BigCommerce Integration Guide
Who should read this:
Any store admin with access to the BigCommerce admin portal.
Global Tracking Script
Place script on every page in header tag (if not already done). Replace the "sid=" parameter value with your store id.
<script type="text/javascript" src="https://cdn.shoppinggives.com/cc-utilities/sgloader.js?sid=XXXXXXXXXX&test-mode=false"></script>
PDP Page (Product Page)
The following code is an example of the product widget integration placed in the base.html file in your Big Commerce store. The bulk of this code is Big Commerce specific code for retrieving and updating of products for the widget. It's main purpose is to feed data into this ShoppingGives function "window.sgProductControllers[0].setSubitem(id, price)" as products are loaded and changed. Replace the "sid=" parameter value on the script with your store id.
<!---------------------------------- SG PRODUCT WIDGET START --------------------------------------------->
{{#if page_type '===' 'product'}}
// PRODUCT WIDGET SCRIPT
<script src="https://cdn.shoppinggives.com/cc-utilities/compact-product.js?sid=**** STORE ID ****&target-element=#form-action-addToCart&after=true"></script>
// SCRIPT TO HANDLE RETRIEVAL AND UPDATING OF PRODUCTS
<script>
// EVENT LISTENER FOR PRODUCT OPTION CHANGES
let addEventHandler = function(elem, eventType, handler) {
if (elem.addEventListener)
elem.addEventListener (eventType, handler, false);
else if (elem.attachEvent)
elem.attachEvent ('on' + eventType, handler);
}
// GETS ALL PRODUCT VARIANTS
let getAllVariants = function(callback) {
let req = new XMLHttpRequest();
let allVariants = {};
req.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
let rawJson = JSON.parse(this.responseText);
for (var i = 0; i < rawJson.data.site.products.edges.length; i++) {
for (var index = 0; index < rawJson.data.site.products.edges[i].node.variants.edges.length; index++) {
allVariants[rawJson.data.site.products.edges[i].node.variants.edges[index].node.entityId] = {
id : rawJson.data.site.products.edges[i].node.variants.edges[index].node.entityId,
price: rawJson.data.site.products.edges[i].node.variants.edges[index].node.prices.price.value
};
}
}
callback(allVariants);
}
}
req.open("POST", "/graphql");
req.setRequestHeader('Authorization', 'Bearer {{ settings.storefront_api.token }}');
req.setRequestHeader('content-type', 'application/json');
req.send(JSON.stringify({
query:`
query SingleProduct {
site {
products (entityIds : [{{ product.id }}]) {
edges {
node {
sku
variants(first: 100) {
edges {
node {
entityId
sku
prices {
price {
value
}
salePrice {
value
}
}
}
}
}
}
}
}
}
}
`
}));
}
// GETS CURRENTLY SELECTED PRODUCT VARIANT
let getVariantId = function(callback) {
d = [];
document.querySelectorAll('form[action$="/cart.php"] input[name^="attribute"]:checked,form[action$="/cart.php"] select[name^="attribute"] option:checked').forEach(function(v) {
v.nodeName === "OPTION" ? d.push(v.parentElement.getAttribute("name") + "=" + v.value) : d.push(v.getAttribute("name") + "=" + v.value)
});
var req = new XMLHttpRequest();
req.onreadystatechange = function(){
// todo - handle errors
if (this.readyState == 4 && this.status == 200) {
callback(JSON.parse(this.responseText).data.v3_variant_id);
}
};
let product_id = document.querySelector('input[name="product_id"]').value;
req.open("POST", "//" + location.hostname + "/remote/v1/product-attributes/" + product_id);
req.setRequestHeader('x-xsrf-token', BCData && BCData.csrf_token ? BCData.csrf_token : "");
req.setRequestHeader('content-type', 'application/x-www-form-urlencoded');
params = "action=add&qty[]=1&product_id=" + product_id + "&" + d.join("&");
req.send(params);
}
// UPDATES WIDGET WITH CURRENT VARIANTS
let refreshWidget = function() {
getVariantId(function(currentVariantId) {
if (!currentVariantId) {
window.sgProductControllers[0].setSubitem(window.allVariants[Object.keys(window.allVariants)[0]].id,(window.allVariants[Object.keys(window.allVariants)[0]].price));
return;
}
console.log(window.allVariants)
window.sgProductControllers[0].setSubitem(window.allVariants[currentVariantId].id,(window.allVariants[currentVariantId].price));
});
}
// MAIN FUNCTION FOR LOADING PDP WIDGET
window.onload = function() {
getAllVariants(function(variants) {
window.allVariants = variants;
refreshWidget();
addEventHandler(document.querySelector('[data-product-option-change]'), 'change', function() {
refreshWidget();
});
});
}
</script>
{{/if}}
<!------------------------------------- SG PRODUCT WIDGET END ----------------------------------------------------->
Cart Widget
The following code is an example of the cart integration placed in the base.html file in your Big Commerce store. It retrieves the cart's content and passes the data in to the cart widget. Replace the "sid=" parameter value on the script with your store id.
<!------------------------------------- SG CART WIDGET START ------------------------------------------------------>
{{#if page_type '===' 'cart'}}
<script src="https://cdn.shoppinggives.com/cc-utilities/contained-cart.js?sid=*** STORE ID ***&target-element=.cart-actions&before=true"></script>
<script>
// FETCH CART CONTENTS
fetch('/api/storefront/cart', {
credentials: 'include'
}).then(function(response) {
return response.json();
}).then(function(json) {
window.cc_cart_items = [];
// PHYSICAL ITEMS
for (var i=0; i < json[0].lineItems.physicalItems.length; i++) {
let item = json[0].lineItems.physicalItems[i];
window.cc_cart_items.push({ id: item.variantId, price: item.listPrice, quantity: item.quantity, discount: Math.abs(item.couponAmount) + Math.abs(item.discountAmount) });
}
// CUSTOM ITEMS
for (var i=0; i < json[0].lineItems.customItems.length; i++) {
let item = json[0].lineItems.customItems[i];
window.cc_cart_items.push({ id: item.variantId, price: item.listPrice, quantity: item.quantity, discount: Math.abs(item.couponAmount) + Math.abs(item.discountAmount) });
}
// DIGITAL ITEMS
for (var i=0; i < json[0].lineItems.digitalItems.length; i++) {
let item = json[0].lineItems.digitalItems[i];
window.cc_cart_items.push({ id: item.variantId, price: item.listPrice, quantity: item.quantity, discount: Math.abs(item.couponAmount) + Math.abs(item.discountAmount) });
}
// GIFT CERTIFICATES
for (var i=0; i < json[0].lineItems.giftCertificates.length; i++) {
let item = json[0].lineItems.giftCertificates[i];
window.cc_cart_items.push({ id: item.variantId, price: item.listPrice, quantity: item.quantity, discount: Math.abs(item.couponAmount) + Math.abs(item.discountAmount) });
}
// REFRESH CART WIDGET
window.sgCartControllers[0].refreshCart();
});
</script>
{{/if}}
<!------------------------------------- SG CART WIDGET END ------------------------------------------------------>
Create API Key
- In your BigCommerce admin, go to: Advanced Settings -> API Accounts
- Click Create API Account -> Create V2/V3 API Token
- Name it โChangeCommerceโ (without the quotes)
- Under OAuth Scopes: Set Orders and Order Transactions to Read-Only, the rest can be left as none
- A text .txt file will download, email to [email protected] as an attachment
Add Post-Conversion Script
- In your BigCommerce admin, go to: Advanced Settings -> Web Analytics
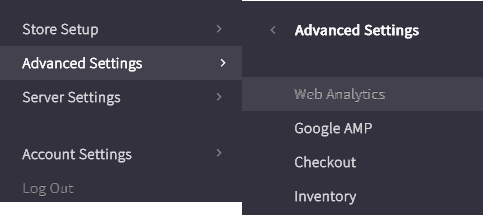
- Make sure that Affiliate Conversion Tracking is selected and save
-
Click the Affiliate Conversion Tracking tab
-
Replace storeId with your Shopping Gives Store Id and place the following script tag in the input:
<script src="https://cdn.shoppinggives.com/cc-utilities/conversion.js?sid=**storeId**&oid=%%ORDER_ID%%"></script>
Updated over 1 year ago